Folks, you know what? For a moment, I asked myself, "Why don't I just do this?" As fellow starters, lending a hand to one another is a generous gesture, right? There is one thing you should consider before we begin. It is helpful for whatever language you choose to code in to be a compiler language. As the saying goes, after you've studied a good deal of interpreter language, you won't be bothered by anything about a compiler language. Go ahead if such feelings don't exist but if not, start with feeling at ease with the latter. By the way, like tada, "Data" can be very insightful if you are analytical! Some glimpses into an interesting course I explored, along with other basic undertakings.
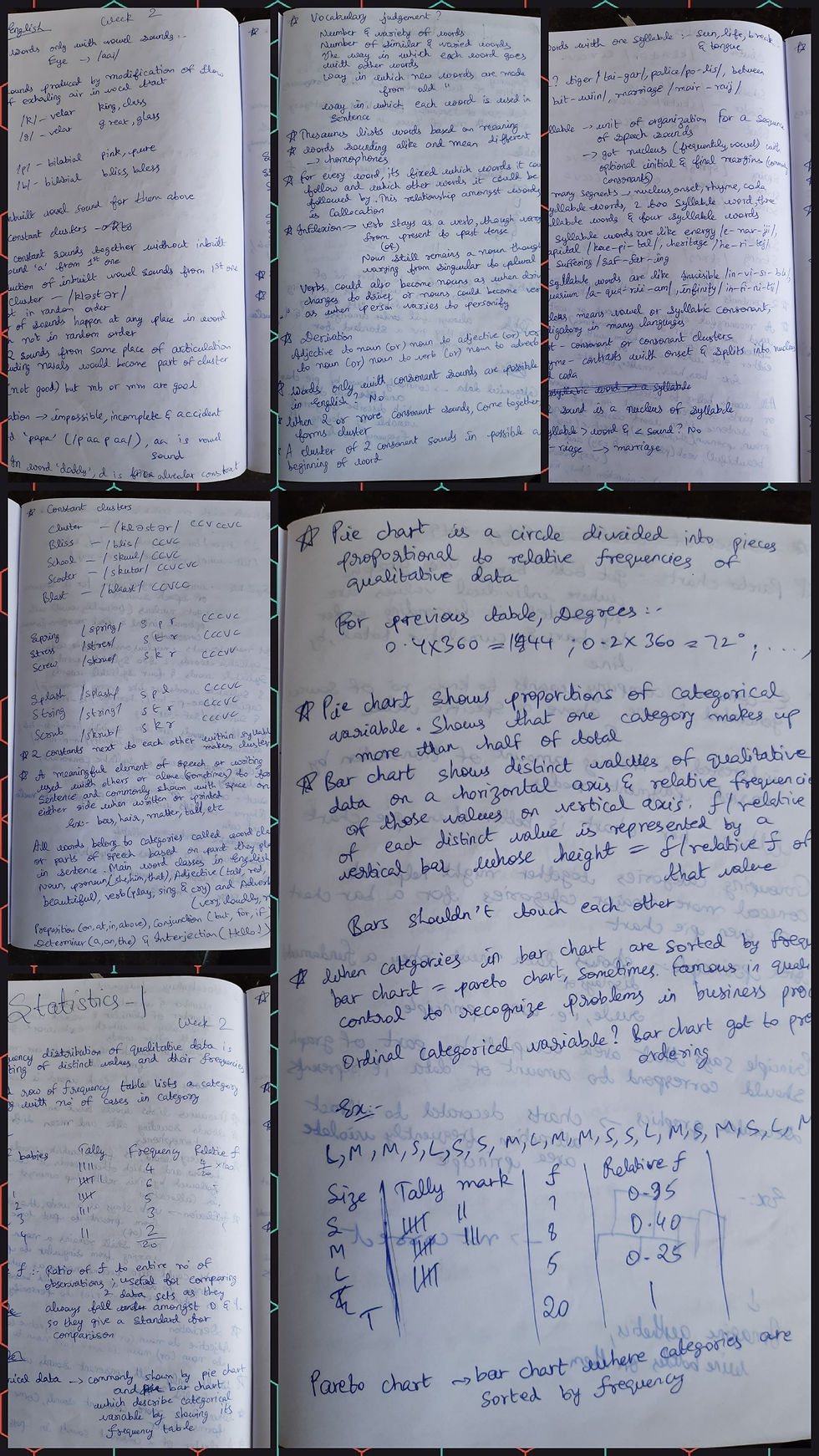
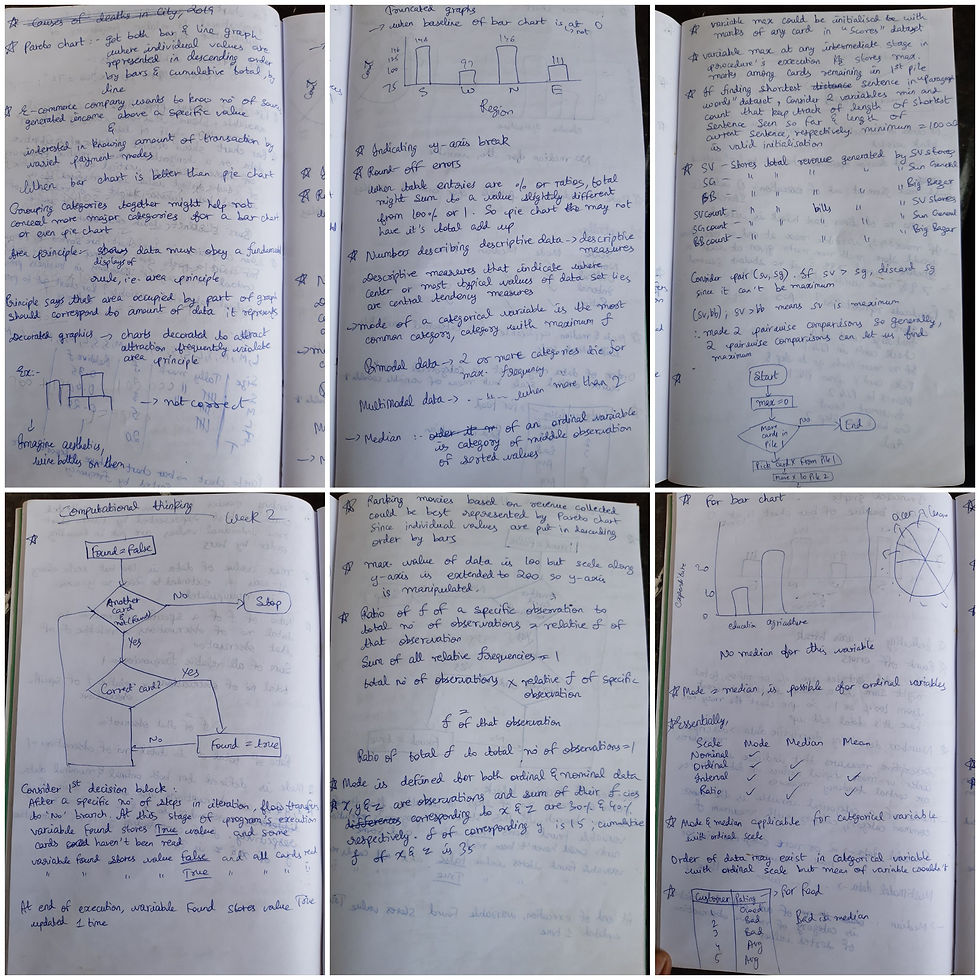
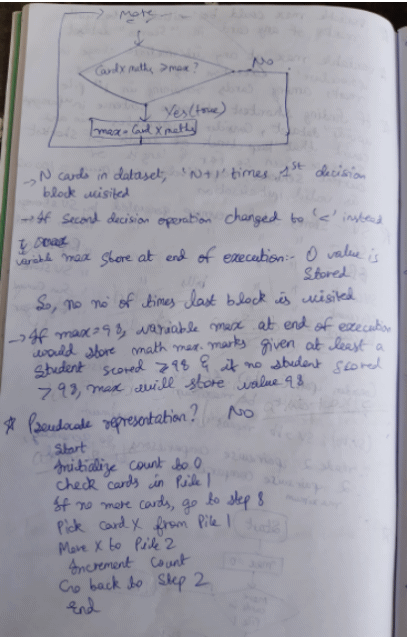
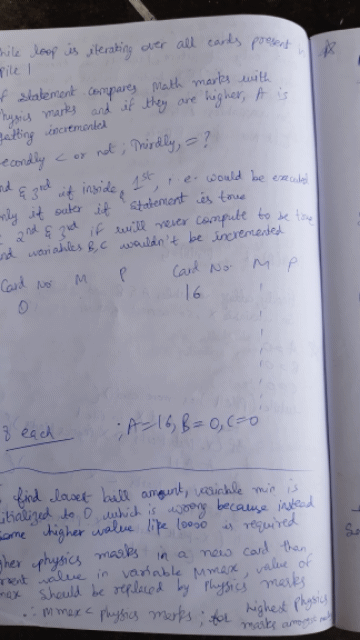
Warming-up more than you thought you may like to
Display "Happy to see you after a long long time through screens!" on the screen
#include<stdio.h>
int main()
{
printf("Happy to see you after a long long time through screens!");
return 0;
}
O/P
Happy to see you after a long long time through screens
Display a name and traits on different lines
#include<stdio.h>
int main()
{
printf("Bhaskari\nDeeply analytical with a poetic edge");
return 0;
}
O/P
Bhaskari
Deeply analytical with a poetic edge
Calculate the area of a circle using Area = PI * r * r
#include<stdio.h>
int main()
{
int r ;
float PI = 3.141592654;
float A;
printf("Enter the value of radius:");
scanf("%d",&r);
A = PI * r * r;
printf("Area of a circle is %f\n",A);
return 0 ;
}
O/P
Enter the value of radius:2
Area of a circle is 12.566371
Calculate the area and volume of a sphere. Consider PI
#include<stdio.h>
int main()
{
int r ;
float PI = 3.141592654;
float A;
float V;
printf("Enter the value of radius:");
scanf("%d",&r);
A = 4 * PI * r * r;
printf("Area of sphere is %f\n",A);
V = 4/3 * PI * r * r * r;
printf("Volume of sphere is %f\n",V);
return 0;
}
O/P
Enter the value of radius:2
Area of sphere is 50.265484
Volume of sphere is 25.132742
Print the product of any 2 numbers from user
#include<stdio.h>
int main()
{
int num1, num2 ;
int product;
printf("Enter the first number:");
scanf("%d",&num1);
printf("Enter the next number:");
scanf("%d",&num2);
product = num1 * num2;
printf("Product: %d\n",product);
return 0;
}
O/P
Enter the first number:9
Enter the next number:8
Product: 72
Convert Celsius C to Fahrenheit F. Consider C= (F-32)/1.8
Do F to C also
#include<stdio.h>
int main()
{
float C ;
float F ;
printf("Enter a value for temperature in Celsius:");
scanf("%f",&C);
F = C*1.8 + 32;
printf(" %f in Celsius = %f\n",C,F);
/* Need to specify kind of data that each of the variable is. The thing is that you would be doing C to F, so type C and then, F */
printf("Enter a value for temperature in Fahrenheit:");
scanf("%f",&F);
C= (F-32)/1.8;
printf("Temperature %f in Fahrenheit=%f\n",F,C);
return 0;
}
O/P
Enter a value for temperature in Celsius:37
37.000000 in Celsius = 98.599998
Enter a value for temperature in Fahrenheit:130
Temperature 130.000000 in Fahrenheit=54.444443
Convert time in seconds to H:M:S style
#include<stdio.h>
int main()
{
int h;
int m;
int s;
int t;
printf("Enter the number of seconds in question:");
scanf("%d",&t);
h = t/3600;
m = (t - 3600*h) / 60;
s = t - 3600*h - 60*m;
printf("t in h:m:s is %d:%d:%d \n", h , m , s);
return 0;
}
O/P
Enter the number of seconds in question:3620
t in h:m:s is 1:0:20
Will the character given by the user be a digit or not, through the conditional operator?
#include<stdio.h>
#include<stdbool.h>
int main()
{
char ch;
char *isDigit;
/* isdigit() function determines if the character passed through the keyboard is a digit or not*/
printf("Enter a character:");
scanf("%c",&ch);
/* Any character is an alphabet
if((ch >= 'a' && ch <= 'z') || (ch >= 'A' && ch <= 'Z'))
|| is OR, a logical operator
Any character is a digit
if(ch >= '0' && ch <= '9')
Otherwise, it's a special symbol
You could consider ASCII codes rather */
isDigit=(ch>='a' && ch<='z')?"Correct":"Incorrect";
/* Checked if the character is an alphabet or not */
printf("is it Digit? : %s \n", isDigit);
return 0;
}
O/P
Enter a character:a
is it Digit? : Correct
Swap variable values of i and j with and without a temporary variable
#include<stdio.h>
int main()
{
int i , j , temp ;
printf("Enter a value of i:");
scanf("%d",&i);
printf("Enter a value of j:");
scanf("%d",&j);
/* You can print the scenario prior to swapping */
/* These are the statements showing how the swapping would work */
temp = i;
i = j;
j = temp;
/* Don't forget to mention the format specifers in printing the needful */
printf("Once swapped, it is i = %d, j = %d \n",i,j);
return 0;
}
O/P
Enter a value of i:3
Enter a value of j:4
Once swapped, it is i = 4 , j = 3
#include<stdio.h>
int main()
{
int i;
int j;
printf("Enter a value of i:");
scanf("%d",&i);
printf("Enter a value of j:");
scanf("%d",&j);
/* Another approach */
i = i+j ;
j = i-j ;
i = i-j ;
printf("Once swapped, it is i = %d, j = %d \n",i,j);
return 0;
}
O/P
Enter a value of i:3
Enter a value of j:4
Once swapped, it is i = 4, j = 3
Find the maximum of three numbers.
#include<stdio.h>
int main()
{
int num1,num2,num3;
int greatest;
printf("Enter the 1st number:");
scanf("%d",&num1);
printf("Enter the 2nd number:");
scanf("%d",&num2);
printf("Enter the 3rd number:");
scanf("%d",&num3);
if (num1 > num2) {
greatest = num1;
}
else {
greatest = num2;
}
if (num2 > num3) {
greatest = num2;
}
else {
greatest = num3;
}
if (num3 > num1) {
greatest = num3;
}
else {
greatest = num1;
}
printf("Greatest number of all : %d \n",greatest);
return 0;
}
O/P
Enter the 1st number:29
Enter the 2nd number:789
Enter the 3rd number:987
Greatest number of all : 987
Class average problem with counter- controlled repetition.
#include <stdio.h>
int main()
{
int n;
int i;
int num;
float sum=0;
float avg;
printf("Enter the number of entries:");
scanf("%d",&n);
for (i=1 ; i<=n ; i++)
{
printf("Enter entry %d:",i);
scanf("%d",&num);
sum = sum + num;
}
avg = sum/n;
printf("\n Average of those %d numbers : %f \n",n,avg);
return 0;
}
O/P
Enter the number of entries:3
Enter entry 1:9
Enter entry 2:8
Enter entry 3:7
Average of those 3 numbers : 8.000000
Class average problem with sentinel-controlled repetition.
#include <stdio.h>
int main()
{
int count=0;
int num;
float sum=0;
float avg;
char ch;
while(1)
{
count=count+1;
printf("\nEnter entry %d:",count);
scanf("%d",&num);
sum = sum+num;
printf("\nWill you add another entry? type (Y) for Yes, type (N) for No:");
scanf("%c",&ch);
if ((ch=='N')||(ch=='n'))
{
break;
}
}
avg=sum/count;
printf("\n Average of above %d numbers are equals to:%f\n",count,avg);
return 0;
}
O/P
Enter entry 1:1
Will you add another entry? type (Y) for Yes, type (N) for No:
Enter entry 2:2
Will you add another entry? type (Y) for Yes, type (N) for No:
Enter entry 3:3
Will you add another entry? type (Y) for Yes, type (N) for No:
Enter entry 4:4
Will you add another entry? type (Y) for Yes, type (N) for No:
Enter entry 5:5
Will you add another entry? type (Y) for Yes, type (N) for No:
Enter entry 6:N
Will you add another entry? type (Y) for Yes, type (N) for No:
Average of above 6 numbers are equals to:3.333333
Figure out whether an input number is Odd or Even.
#include<stdio.h>
#include<stdlib.h>
int main()
{
int val;
printf("Enter a value:");
scanf("%d",&val);
/* if the value is divided by 2, then you should get 0 for an even number but if not, this would be an odd number */
if (val%2==0)
{
printf("This is an even number\n");
}
else {
printf("It's an odd number\n");
}
return 0;
}
O/P
Enter a value:2
This is an even number
Create a calculator using menu driven Program.
#include<stdio.h>
#include<stdlib.h>
int main()
{
int op1,op2,sum,diff,mul,div;
int ch;
while(1)
{
printf("\n---Menu---");
printf("\n1.Addition\n2.Subtraction\n3.Multiplication\n4.Division\n5.Exit\n");
printf("\nEnter your operation:");
scanf("%d",&ch);
if (ch<5) {
printf("Enter 1st operand:");
scanf("%d",&op1);
printf("Enter 2nd operand:");
scanf("%d",&op2);
}
switch(ch)
{
case 1: sum=op1+op2;
printf("\n Sum of %d and %d is %d \n",op1,op2,sum);
break;
case 2:diff=op1-op2;
printf("\n Difference of %d and %d is %d \n",op1,op2,diff);
break;
case 3:mul=op1*op2;
printf("\n Multiplication of %d and %d is %d \n",op1,op2,mul);
break;
case 4:if(op2==0)
{
printf("\nDivision of %d by %d is undefined\n",op1,op2);
}
else {
div = op1/op2;
printf("\nDivision of %d by %d is %d\n",op1,op2,op1/op2);
}
break;
case 5:printf("\n Done \n");
exit(1);
default:printf("\n Opt for the right operator now \n");
break;
}
}
return 0;
}
O/P
/tmp/RIH6IiIkP0.o
---Menu---
1.Addition
2.Subtraction
3.Multiplication
4.Division
5.Exit
Enter your operation:3
Enter 1st operand:9
Enter 2nd operand:6
Multiplication of 9 and 6 is 54
---Menu---
1.Addition
2.Subtraction
3.Multiplication
4.Division
5.Exit
Enter your operation:
Convert decimal to binary.
#include<stdio.h>
int main()
{
int num, rem;
char binary [20];
int count=0;
int temp;
printf("Enter a value:");
scanf("%d",&num);
temp = num;
while (num>0) {
rem = num%2;
binary[count]=48+rem;
count=count+1;
num=num/2;
}
printf("\n Binary form of %d is:",temp);
for(int i=count-1;i>=0;i--)
{
printf("%c",binary[i]);
}
return 0;
}
O/P
Enter a value:3
Binary form of 3 is:11
Convert decimal to hexadecimal.
#include<stdio.h>
int main()
{
int val, rem, temp;
char hexadecimal [20];
int count = 0;
printf("Enter a value:");
scanf("%d",&val);
temp=val;
while(val>0) {
rem=val%16;
if (rem<10)
{
hexadecimal[count]=48+rem;
}
else {
hexadecimal[count]=65+(rem-10);
}
count=count+1;
val=val/16;
}
printf("\n Hexadecimal form of %d is:",temp);
for(int i=count-1;i>=0;i--)
{
printf("%c",hexadecimal[i]);
}
return 0;
}
O/P
Enter a value:25
Hexadecimal form of 25 is:19
Find the sum of the first 100 natural numbers.
#include<stdio.h>
int main()
{
int i;
int sum=0;
for (i=1;i<=100;i++) {
sum=sum+i;
}
printf("Sum of 1st 100 natural numbers is = %d",sum);
return 0;
}
O/P
Sum of 1st 100 natural numbers is = 5050
Find the sum of odd numbers and even numbers between 1 to n
#include<stdio.h>
int main()
{
int i,num;
int sum=0;
printf("Enter the upper limit:");
scanf("%d",&num);
for (i=1;i<=2*num;i++) {
if (i%2==1)
{
sum=sum+i;
}
}
printf("Sum of 1st %d odd numbers is = %d\n",2*num,sum);
return 0;
}
O/P
Enter the upper limit:20
Sum of 1st 40 odd numbers is = 400
#include<stdio.h>
int main()
{
int i,num;
int sum=0;
printf("Enter the upper limit:");
scanf("%d",&num);
for (i=1;i<=2*num;i++) {
if (i%2==0)
{
sum=sum+i;
}
}
printf("Sum of 1st %d even numbers is = %d\n",num,sum);
return 0;
}
O/P
Enter the upper limit:30
Sum of 1st 30 even numbers is = 930
Display the first 100 prime numbers.
#include<stdio.h>
int main()
{
int i,n=1;
int count=1;
int fac=0;
while(count<=100) {
fac=0;
for(i=1;i<=n;i++)
{
if (n%i==0)
{
fac=fac+1;
}
}
if (fac==2) {
printf("Prime %d:%d\n",count,n);
count++;
}
n++;
}
return 0;
}
O/P
Prime 1:2
Prime 2:3
Prime 3:5
Prime 4:7
Prime 5:11
Prime 6:13
Prime 7:17
Prime 8:19
Prime 9:23
Prime 10:29
Prime 11:31
Prime 12:37
Prime 13:41
Prime 14:43
Prime 15:47
Prime 16:53
Prime 17:59
Prime 18:61
Prime 19:67
Prime 20:71
Prime 21:73
Prime 22:79
Prime 23:83
Prime 24:89
Prime 25:97
Prime 26:101
Prime 27:103
Prime 28:107
Prime 29:109
Prime 30:113
Prime 31:127
Prime 32:131
Prime 33:137
Prime 34:139
Prime 35:149
Prime 36:151
Prime 37:157
Prime 38:163
Prime 39:167
Prime 40:173
Prime 41:179
Prime 42:181
Prime 43:191
Prime 44:193
Prime 45:197
Prime 46:199
Prime 47:211
Prime 48:223
Prime 49:227
Prime 50:229
Prime 51:233
Prime 52:239
Prime 53:241
Prime 54:251
Prime 55:257
Prime 56:263
Prime 57:269
Prime 58:271
Prime 59:277
Prime 60:281
Prime 61:283
Prime 62:293
Prime 63:307
Prime 64:311
Prime 65:313
Prime 66:317
Prime 67:331
Prime 68:337
Prime 69:347
Prime 70:349
Prime 71:353
Prime 72:359
Prime 73:367
Prime 74:373
Prime 75:379
Prime 76:383
Prime 77:389
Prime 78:397
Prime 79:401
Prime 80:409
Prime 81:419
Prime 82:421
Prime 83:431
Prime 84:433
Prime 85:439
Prime 86:443
Prime 87:449
Prime 88:457
Prime 89:461
Prime 90:463
Prime 91:467
Prime 92:479
Prime 93:487
Prime 94:491
Prime 95:499
Prime 96:503
Prime 97:509
Prime 98:521
Prime 99:523
Prime 100:541
Figure out the sum of digits of any accepted number.
#include<stdio.h>
int main()
{
int n;
int sum=0;
int rem;
printf("Enter the number:");
scanf("%d",&n);
while(n>0) {
rem=n%10;
sum=sum+rem;
n=n/10;
}
printf("\nSum of digits of accepted numbers is:%d\n",sum);
return 0;
}
O/P
Enter the number:12332
Sum of digits of accepted numbers is:11
Check if the given number is palindrome or not.
#include<stdio.h>
int main() {
int n,rem;
int res=0;
int temp;
printf("Enter a number:");
scanf("%d",&n);
temp=n;
while(n>0) {
rem=n%10;
res=res*10+rem;
n=n/10;
}
if(res=temp) {
printf("\nThis is a palindrome");
}
else {
printf("\nThis is not a palindrome");
}
return 0;
}
O/P
Enter a number:3
This is a palindrome
Print the number in digits.
#include<stdio.h>
int main()
{
int n,rem;
int digits[20];
int count=0;
printf("Enter the number:");
scanf("%d",&n);
while(n>0) {
rem=n%10;
digits[count]=rem;
count=count+1;
n=n/10;
}
for(int i=count-1;i>=0;i--)
{
switch(digits[i])
{
case 0:printf("zero\n");break;
case 1:printf("one\n");break;
case 2:printf("two\n");break;
case 3:printf("three\n");break;
case 4:printf("four\n");break;
case 5:printf("five\n");break;
case 6:printf("six\n");break;
case 7:printf("seven\n");break;
case 8:printf("eight\n");break;
case 9:printf("nine\n");break;
default:printf("Invalid digit");break;
}
}
return 0;
}
O/P
Enter the number:982347
nine
eight
two
three
four
seven
Comments